Database Tuning: Techniques to Boost Performance and Scalability
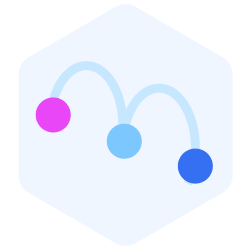
Database Tuning: Techniques to Boost Performance and Scalability
What is Database Tuning?
Database tuning is the process of optimizing a database to improve its performance, efficiency, and reliability. It is used to identify and resolve bottlenecks, optimize query execution, refine database structures, and adjust system configurations to operate smoothly under various workloads. Database tuning aims to enhance query speed, reduce resource consumption, and ensure scalability as data volumes and user demands grow.
While traditional SQL databases focus on structured data, NoSQL databases are designed for unstructured and semi-structured data, and vector databases like Milvus manage high-dimensional vector data in AI and machine learning applications. Tuning applies to all these systems, with tailored strategies depending on the database type.
Why Database Performance Matters in Modern Applications?
Speed is everything in today’s digital world. Whether it’s an e-commerce site processing orders or a social media app loading your feed, users expect instant results. Databases are the backbone of these applications; if they’re slow, the entire app feels sluggish. This frustrates users, leading to abandoned carts, negative reviews, or even switching to competitors, which ultimately damages trust and brand reputation.
Even minor delays can have a significant business impact. Studies show that a few extra seconds can hurt user retention and sales. For modern applications to scale with growing data and users, databases must handle increased demand without crashing. Database tuning is essential to keep apps running smoothly, improve user satisfaction, and help businesses stay competitive in a fast-paced, data-driven world.
Overview of Different Database Types
Modern databases are designed to cater to different data needs and workloads. Understanding their differences is crucial before exploring tuning techniques, as each type requires unique optimization strategies. Below is an overview of the most common database types:
SQL Databases: Relational databases like MySQL, PostgreSQL, and SQL Server manage structured data with predefined schemas. They are widely used for transactional workloads and applications requiring strong data consistency.
NoSQL Databases: These databases, such as MongoDB and Cassandra, handle unstructured or semi-structured data. NoSQL databases are highly scalable and support flexible data models, making them suitable for real-time applications, large-scale analytics, and distributed systems.
Vector databases: Specialized systems like Milvus are designed to store and search high-dimensional vector data known as embeddings generated by AI and machine learning models. These databases power applications like semantic search, recommendation systems, and anomaly detection.
Key Components of Database Performance
The performance of a database depends on several key factors that determine how efficiently it handles queries, manages resources, and scales with demand. For example:
Query Execution Speed: The time it takes for the database to process and return results for a query. Faster execution means quicker responses for applications and users. In vector databases, execution speed is determined by the efficiency of vector comparisons and search algorithms.
Storage Efficiency: Storing data in a way that reduces unnecessary space usage while keeping the data easy to retrieve. Efficient storage speeds up data access and minimizes storage costs.
Scalability: The ability of the database to grow with the application, handling more users or larger datasets without slowing down or breaking.
Resource Utilization: Balancing CPU, memory, and disk I/O to prevent bottlenecks. Overloading any one resource can cause the entire system to lag or crash.
Unlike traditional relational databases, vector databases conduct approximate rather than precise searches, so there are two additional metrics related to performance: indexing construction time and recall rate.
Index construction time: the duration needed to build vector indexes
Recall rate: a metric denoting retrieval accuracy.
Building indexes requires significant computational resources, leading to a trade-off between query accuracy and efficiency. Prioritizing accuracy may affect query speed and vice versa. Therefore, balancing both aspects is vital rather than focusing solely on latency and query speed.
Common Database Performance Bottlenecks
Several factors can contribute to a database's performance bottlenecks that impact its efficiency and reliability. For example:
Slow Queries: Complex or poorly written queries or searching algorithms take longer to execute, straining the database and delaying users' results.
Inefficient Indexing: A lack of indexes or too many unnecessary indexes can slow down data retrieval, as the database has to scan more rows than needed.
Locking and Contention: When multiple processes try to access or update the same data simultaneously, it can cause delays or even deadlocks that block other operations.
Poor Schema Design: Badly structured tables or collections, such as suboptimal partitioning or grouping of vectors, can lead to slower searches, redundant computations, or unnecessary complexity in managing data relationships.
Data Overhead: Old, unused, or redundant data increases the database's size, increasing the query times and storage costs.
Larger Dataset Size and Higher Vector Dimensionality: for vector databases, the vector size and dimensionality also profoundly influence their performance. Larger datasets with higher vector dimensionality usually present more formidable challenges to vector databases' distributed architecture, leading to decreased performance.
Database Tuning Techniques
Database tuning involves various techniques to optimize performance, scalability, and resource utilization. Whether dealing with SQL, NoSQL, or vector databases, these techniques address specific bottlenecks and improve efficiency.
Here are some commonly used strategies for database tuning:
1. Query Optimization
Efficient queries are the foundation of database performance. Poorly written queries can slow down the entire system, while optimized queries improve speed and reduce resource usage.
- For SQL Databases: Simplify complex queries by breaking them into smaller, more efficient steps. Avoid using
SELECT *
, which fetches unnecessary columns, and instead specify only the required fields.
-- Inefficient query
SELECT * FROM employees;
-- Optimized query
SELECT id, name, position FROM employees;
Analyze queries using tools like EXPLAIN
to understand execution plans and identify bottlenecks:
EXPLAIN SELECT name FROM employees WHERE department_id = 10;
For Vector Databases:Optimize vector search parameters to balance speed and accuracy. For example, in Milvus:
nprobe: Controls the number of clusters searched in IVF indices. Increasing nprobe improves recall but increases latency.
ef: Determines the size of the candidate list in HNSW. A higher ef improves search accuracy but uses more memory.
Code Example:
# Milvus example: Optimize search parameters
search_params = {"metric_type": "L2", "params": {"nprobe": 10}}
results = collection.search(vectors, "field_name", params=search_params, limit=10)
2. Indexing Strategies
Indexes allow databases to locate data faster, avoiding full table scans. Choosing the right indexing strategy is critical for performance.
For SQL Databases: Use single-column indexes for basic lookups and composite indexes for multiple-column queries.
Example:
-- Single-column index
CREATE INDEX idx_department_id ON employees(department_id);
-- Composite index
CREATE INDEX idx_name_department ON employees(name, department_id);
Regularly rebuild or optimize indexes to maintain their efficiency:
REINDEX TABLE employees;
For Vector Databases: Select an appropriate index type based on the use case:
HNSW (Hierarchical Navigable Small World): Fast for approximate nearest neighbour searches.
IVF_FLAT (Inverted File with Flat): Suitable for precise searches but slower for large datasets.
Milvus supports various index types, including IVF_FLAT, HNSW, FAISS, and ANNOY, each of which affects performance differently.
Example in Milvus:
# Create an HNSW index in Milvus
index_params = {"index_type": "HNSW", "metric_type": "COSINE", "params": {"M": 16, "efConstruction": 500}}
collection.create_index(field_name="vector_field", index_params=index_params)
3. Schema or Collection Design
Efficient data organization reduces complexity and improves query performance.
- For SQL Databases: Normalize schemas to reduce redundancy and save storage, but denormalize when read performance outweighs the need for space savings.
Example:
-- Normalized schema: Separate tables for customers and orders
SELECT orders.id, customers.name
FROM orders
JOIN customers ON orders.customer_id = customers.id;
-- Denormalized schema: Faster read with redundancy
SELECT id, customer_name FROM orders;
- For Vector Databases: Group similar vectors into logical partitions (e.g., by category or time) to improve search performance. Partitioning ensures that queries access only relevant subsets of data.
Example:
# Create a partition
collection.create_partition(partition_name="category_A")
# Insert data into the partition
collection.insert(data=[ids, categories, vectors], partition_name="category_A")
# Search within a specific partition
results = collection.search(
data=search_vectors,
anns_field="embedding",
param={"metric_type": "L2", "params": {"nprobe": 10}},
limit=3,
partition_names=["category_A"] # Restrict search to this partition
)
4. Caching Mechanisms
Caching reduces the need for repeated computations by storing frequently accessed data in memory.
- For SQL and NoSQL Databases: Use external tools like Redis or Memcached to cache query results. Example in Python:
import redis
cache = redis.Redis(host='localhost', port=6379, db=0)
result = cache.get("recent_orders")
if not result:
result = db.query("SELECT * FROM orders WHERE date > NOW() - INTERVAL '1 day'")
cache.set("recent_orders", result, ex=3600) # Cache for 1 hour
- For Vector Databases: Cache frequently searches embeddings or query results to reduce redundant computations. This is especially useful for AI applications with repeated similarity searches. Milvus implements caching mechanisms to improve query performance.
Example:
from cachetools import LRUCache
# Initialize an LRU cache to store query results
cache = LRUCache(maxsize=100) # Cache up to 100 results
def search_with_cache(collection, search_vectors, cache_key):
if cache_key in cache:
return cache[cache_key] # Return cached results
# Perform the search
results = collection.search(
data=search_vectors,
anns_field="embedding",
param={"metric_type": "L2", "params": {"nprobe": 10}},
limit=5
)
# Cache the results
cache[cache_key] = results
return results
# Example usage
cache_key = "vector_search_1" # Unique key for this query
results = search_with_cache(collection, search_vectors, cache_key)
5. Resource Management
Efficient resource allocation ensures the database can handle workloads smoothly without bottlenecks.
- For SQL Databases: Allocate memory for frequently accessed data (e.g., increasing buffer pool size in MySQL):
SET GLOBAL innodb_buffer_pool_size = 1GB;
- For Vector Databases: Utilize GPUs for computationally intensive tasks like vector similarity searches, as they can significantly reduce query latency. Adjust memory and disk I/O allocation to prevent resource contention.
collection.load(load_param={"use_gpu": True}) # Enable GPU usage for search
6. Partitioning and Sharding
Partitioning and sharding improve scalability by dividing large datasets into smaller, more manageable segments.
- For SQL and NoSQL Databases: Partition data based on logical criteria, such as date ranges or regions.
Example:
CREATE TABLE sales (
id SERIAL PRIMARY KEY,
sale_date DATE NOT NULL,
amount NUMERIC
) PARTITION BY RANGE (sale_date);
CREATE TABLE sales_2023 PARTITION OF sales
FOR VALUES FROM ('2023-01-01') TO ('2024-01-01');
- For Vector Databases: Shard large datasets across multiple nodes to distribute the workload evenly. Use partitioning to group related vectors for faster search. Milvus supports partitioning and sharding to enhance scalability and performance and for load balancing.
Example:
# Create a partition for related vectors
collection.create_partition(partition_name="category_A")
# Load a specific partition on a node for efficient search
collection.load(partition_names=["category_A"], replica_number=2) # Distribute workload across 2 nodes
7. Monitoring
Monitoring database performance is essential for identifying bottlenecks, analyzing query performance, and optimal resource utilization. Monitoring applies to SQL, NoSQL, and vector databases, with tailored strategies for each.
- For SQL Databases:
Use built-in tools like pg_stat_activity
(PostgreSQL) or Performance Schema
(MySQL) to track query latency, resource utilization, and lock contention.
Example: Monitor slow query logs to identify inefficient queries:
SET GLOBAL slow_query_log = 'ON';
SET GLOBAL long_query_time = 1; -- Log queries taking longer than 1 second
- For NoSQL Databases:
Monitor throughput, latency, and consistency issues. Tools like MongoDB Atlas provide real-time insights into operations.
Example Metric: Use MongoDB’s db.currentOp()
to monitor long-running operations:
db.currentOp({ secs_running: { $gte: 5 } }) // Find operations running for 5+ seconds
- For Vector Databases:
Monitor metrics like:
Query latency: Time taken for vector similarity searches.
Indexing time: Efficiency of index creation and updates.
Resource utilization: CPU, GPU, and memory usage during searches.
Use tools like Prometheus and Grafana to track performance in Milvus, integrating with its built-in metrics endpoints.
Example: Track average query latency:
# Use Prometheus to scrape Milvus metrics
http_requests_total{job="milvus-query"} # Example PromQL query
To read more about how to optimize the performance of Milvus, you can dive deeper into this article:
An Overview of Milvus Storage System and Techniques to Evaluate and Optimize Its Performance
How to Spot Search Performance Bottleneck in Vector Databases - Zilliz Learn
Benchmark Vector Database Performance: Techniques & Insights - Zilliz Learn
Challenges of Database Tuning
While database tuning provides significant benefits, it also comes with challenges that require careful consideration and expertise to overcome:
Requires Expertise: Tuning databases demands a deep understanding of database systems, query optimization, indexing, and resource management, which can be challenging for less experienced teams.
Time-Intensive for Large Databases: Analyzing and optimizing large or complex databases takes significant time and effort, especially when dealing with numerous queries and large datasets.
Risk of New Issues: Poorly implemented tuning changes can introduce new problems, such as unexpected query failures or performance regressions.
Dependent on Application Design: Even a perfectly tuned database may not deliver optimal results if the application has poorly written code or inefficient design.
Hardware Limitations: Database tuning can only go so far; performance improvements may be limited if the hardware is outdated or underpowered.
Best Practices for Ongoing Database Maintenance
To ensure long-term database performance and reliability, ongoing maintenance practices are required. For example:
Monitoring and Observability: Implement observability tools to gain real-time insights into database performance. Use dashboards and alerts to track metrics like latency, throughput, and error rates.
Regular Index and Schema Reviews: Evaluate indexes and table structures periodically to align with current usage patterns. Remove unused indexes and optimize schemas as data and application needs evolve.
Periodic Backups and Disaster Recovery Planning: Schedule regular backups and test recovery procedures to safeguard against data loss from system failures or security breaches.
Keep Database Versions Up-to-Date: Upgrade to the latest stable database versions to benefit from performance improvements, bug fixes, and enhanced security features.
Conclusion
Database tuning is vital for fast, reliable, and scalable performance across modern applications, regardless of the database type—SQL, NoSQL, or vector databases. Tuning eliminates bottlenecks that hinder operations by optimizing queries, selecting appropriate indexing strategies, managing resources efficiently, and structuring data thoughtfully. A well-tuned database can handle growing workloads, delivering consistent speed and reliability. Beyond improved performance, tuning enhances user experience, supports scalability, and minimizes operational costs.
FAQs on Database Tuning
- What is database tuning, and why is it important?
Database tuning optimizes various aspects of a database, such as queries, indexing, and resource allocation, to improve performance, scalability, and reliability. It reduces response times, handles large workloads, and enhances user experience.
- What are the common bottlenecks in database performance?
Common bottlenecks include slow queries, inefficient indexing, locking and contention issues, poorly designed schemas, and data overhead from unused or redundant data.
- How can I optimize Milvus for better performance?
To optimize Milvus, select appropriate indices, tune search parameters (e.g., nprobe, ef) to balance speed and accuracy, use partitions to group related vectors, leverage caching for frequently accessed embeddings, and enable GPU acceleration for computationally intensive searches
- How does database tuning benefit modern applications?
Tuning helps applications handle growing workloads, reduces operational costs, and enhances the user experience by improving query speeds, scalability, and overall system efficiency.
- What are the best practices for ongoing database maintenance?
Key practices include monitoring performance with observability tools, reviewing and optimizing indexes and schemas regularly, maintaining backups for disaster recovery, and keeping the database up-to-date with the latest stable versions.
Related Resources
- What is Database Tuning?
- Why Database Performance Matters in Modern Applications?
- Overview of Different Database Types
- Key Components of Database Performance
- Common Database Performance Bottlenecks
- Database Tuning Techniques
- Challenges of Database Tuning
- Best Practices for Ongoing Database Maintenance
- Conclusion
- FAQs on Database Tuning
- Related Resources
Content
Start Free, Scale Easily
Try the fully-managed vector database built for your GenAI applications.
Try Zilliz Cloud for Free