Prometheus Metrics: Monitor Your App's Performance
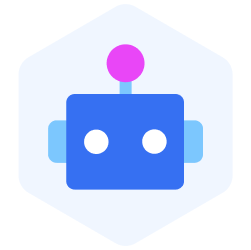
Prometheus Metrics: Monitor Your App's Performance
What is Prometheus?
Prometheus is an open-source tool that tracks the performance and health of software systems. It collects data points, known as metrics, that give insights into how well a system runs. These metrics help detect problems early and analyze system behavior. With Prometheus, teams can spot issues before they impact users to maintain reliable and efficient services.
What are the Metrics in Prometheus?
In Prometheus, metrics are numerical values that track specific aspects of a system's behavior and performance over time. These metrics help understand how well a system functions under various conditions and also provide alerts in conditions that could indicate issues, such as a sudden spike in traffic or a drop in system performance. They can monitor a wide range of data, from the number of active users on a website to the amount of memory an application uses.
Metrics Format in Prometheus
Metrics in Prometheus are stored in a time-series format, with each metric identified by its name and optional key-value pairs called labels, which provide additional context like service names or error types. The primary format for metric data in Prometheus is the Prometheus Exposition Format. This plain text format is easy to generate and parse, consisting of data lines that include the metric name, optional labels, the metric value, and a timestamp.
The Four Types of Metrics in Prometheus
Prometheus categorizes metrics into four main types: counters, gauges, histograms, and summaries. Each type serves a specific function in monitoring and provides different insights into the behavior of your systems.
Counter Metrics
Counter metrics in Prometheus record the number of times a particular event occurs. They only increase over time or reset to zero when the process restarts. Hence, they are used for tracking cumulative quantities like the number of requests handled, tasks completed, or errors logged.
To implement a counter that tracks the number of requests received by a server, you can use the following code snippet. This example sets up a counter to monitor the total number of HTTP requests received. Each time the handle_request
function is called, the counter is incremented.
from prometheus_client import Counter
# Create a counter metric for tracking received requests
request_counter = Counter('http_requests_received_total', 'Total HTTP requests received')
def handle_request(request):
# Process the request
# ...
# Increment the counter by 1 each time this function is called
request_counter.inc()
Best Practices for Using Counters
Reset Awareness: Be aware that counters reset to zero when the process restarts. Design your monitoring to account for these resets.
Use Labels: Use labels with counters to provide more detailed insights, such as distinguishing between different types of errors or requests.
Consistent Incrementing: Make sure that the counter is incremented in the correct place in your code to accurately reflect the events you are tracking.
Monitoring Resets: Use the rate function in queries to calculate the per-second average rate of increase of a counter, which can help you understand trends and detect issues, even with resets.
Gauge Metrics
Gauge metrics in Prometheus measure values that can increase and decrease, such as CPU temperatures, current numbers of running processes, or amounts of free memory. Unlike counters, which only go up, gauges reflect the system's current state at a specific moment in time, making them essential for tracking fluctuating metrics.
To implement a gauge that monitors the amount of free memory in a system, you can use the following code snippet. This example sets up a gauge to monitor free memory, updating the gauge's value by calling the update_free_memory()
function, which should be triggered to reflect system changes.
from prometheus_client import Gauge
# Create a gauge metric to track free memory
free_memory_gauge = Gauge('system_free_memory_bytes', 'Amount of free memory in bytes')
def update_free_memory():
# Assume get_free_memory() is a function that fetches the current free memory
free_memory = get_free_memory()
free_memory_gauge.set(free_memory)
# Update gauge regularly or upon specific system events
update_free_memory()
Tips for Utilizing Gauges
Regular Updates: Verify that gauges are updated regularly to accurately reflect the current state of the system.
Contextual Use: Use gauges for metrics that require tracking rises and falls over time, such as load averages or available system resources.
Avoid Misuse: Be cautious not to use gauges for metrics that should only increment or decrement, where a counter or a histogram might be more appropriate.
Histogram Metrics
Histograms in Prometheus summarize the distribution of numeric data over a set of predefined buckets. Each bucket represents a range of values, and the histogram counts how many values fall into each bucket. This metric type is useful for tracking measurements like request latencies or response sizes, where understanding the distribution can provide more insight than simply knowing the average.
To implement a histogram that tracks the latency of HTTP requests, you can use the following code snippet. This example sets up a histogram with several buckets to measure how long each HTTP request takes to process. The with block automatically measures the duration of the request handling and records it in the appropriate bucket.
from prometheus_client import Histogram
# Define a histogram with buckets for request latency
request_latency_histogram = Histogram('http_request_latency_seconds', 'HTTP request latencies', buckets=[0.1, 0.2, 0.5, 1, 2, 5])
def handle_request(request):
with request_latency_histogram.time():
# Process the request
# This automatically measures the time taken by this block and records it in the histogram
pass
Histogram Buckets and Their Importance
Bucket Design: Choosing the right buckets is crucial for useful histograms. Buckets should be aligned with your application's performance objectives and thresholds. For instance, if you care about differentiating between requests that take 0.1 seconds and 1 second, your buckets should reflect these intervals.
Granularity: More buckets increase the granularity of the histogram but also increase memory usage. Balance detail with resource efficiency.
Cumulative Counting: Prometheus's histograms are cumulative. This means each bucket counts the total number of observations that fall into its range and all previous ranges. This calculates percentiles, which are more informative about data distribution than averages.
Usage in Queries: When querying histograms, functions like histogram
_quantile()
can calculate quantiles from the cumulative buckets, providing powerful insights into your system's performance characteristics.
Summary Metrics
Summary metrics in Prometheus offer a way to calculate quantiles of observations, such as the 90th percentile of request latencies, directly within the client. Unlike histograms, which collect and categorize data into buckets, summaries calculate streaming quantiles without predefined buckets. This makes summaries ideal for situations where precise quantile calculations are needed, particularly when exact thresholds are important, such as reporting on service level agreements (SLAs).
To implement a summary that measures the latency of database queries, you can use the following code snippet. This example sets up a summary to monitor the time database queries take. The with block measures the duration of the query and updates the summary with this new observation, facilitating the calculation of quantiles.
from prometheus_client import Summary
# Create a summary to measure database query latencies
db_query_latency = Summary('db_query_latency_seconds', 'Database query latencies')
def query_database(query):
with db_query_latency.time():
# Execute the database query
# The time taken by this block is automatically recorded and calculated in the summary
pass
Difference Between Histograms and Summaries
Quantile Calculation: Histograms estimate quantiles based on the defined buckets, which can introduce inaccuracies depending on bucket configuration. Summaries calculate quantiles directly from the observed data, potentially offering more precision.
Client-Side Load: Summaries calculate quantiles on the client side, which can increase the computational load, especially with a high number of observations. Histograms, with their pre-defined buckets, can reduce client-side computation.
Configuration: Histograms require prior knowledge of the distribution to set appropriate buckets. Summaries do not require bucket configuration, which makes them easier to deploy initially but potentially more resource-intensive.
Use Cases: Summaries are preferred when accurate, real-time quantiles are needed for critical metrics, while histograms are often better suited for capturing the broader distribution of a metric where exact thresholds are less critical.
Best Practices for Labeling and Grouping Metrics
Appropriate Use of Labels
Labels in Prometheus are key-value pairs that attach metadata to metrics for more detailed and targeted queries. They are important in organizing and identifying metrics across dimensions such as service names, hostnames, or error types. Here are some best practices for using labels:
Descriptive and Consistent: Choose label names that clearly describe their purpose and maintain consistency across your metrics. For example, use service for all metrics that identify the service to which they belong.
Necessary Granularity: While labels can add significant detail to your metrics, too many labels can increase storage costs and decrease query performance. Use labels judiciously to balance granularity with performance.
Avoid High Cardinality: High cardinality labels, such as those that might label every individual user or email address, can increase data size and degrade performance. Stick to labels that have a reasonable number of distinct values.
This example shows a counter that uses labels to distinguish between different HTTP methods and statuses for detailed analysis and monitoring.
from prometheus_client import Counter
# Create a counter with labels for HTTP methods and response statuses
http_requests_total = Counter('http_requests_total', 'Total HTTP requests',
['method', 'status'])
def handle_request(request):
# Increment the counter with the appropriate labels
http_requests_total.labels(method=request.method, status=request.response.status_code).inc()
Strategies for Grouping Metrics
Grouping metrics logically can enhance clarity and improve the performance of monitoring systems. Here are some strategies:
Categorize by Type: Group metrics by type, such as errors, traffic, latency, etc., to make it easier to find and analyze related metrics.
Service-Based Grouping: Organize metrics by the service they measure. This helps in isolating issues within a specific service quickly.
Use Hierarchical Naming: When naming metrics, consider a hierarchical structure that reflects their grouping, such as
service_database_queries_total
orservice_http_requests_total
.
Benefits of Effective Grouping
Improved Query Performance: Logical grouping can lead to more efficient queries by reducing the number of metrics that need to be scanned for each query.
Easier Alert Management: Grouping similar metrics simplifies the creation of alert rules and makes it easier to manage alerts across different parts of your system.
Better Visualization: Grouped metrics are easier to visualize in dashboards, as related metrics can be displayed together, providing a cohesive view of system performance.
Querying Metrics with PromQL
Basics of PromQL and Its Syntax
PromQL, or Prometheus Query Language, is the powerful querying language used by Prometheus to explore data and generate alerts. PromQL allows simple and complex queries to compute the exact data needed from your metrics. The syntax of PromQL supports selecting and aggregating time series data based on metric names, labels, and time intervals.
Key Features of PromQL:
Instant and Range Queries: Instant queries give the current value of the time series for a specific point in time, while range queries return values of the time series for a range of time.
Functions and Operators: PromQL includes various built-in functions and operators for calculating rates, averages, and arithmetic operations between metrics.
Code Snippets: Common Queries for Each Type of Metric
Counter Metrics
This query calculates the per-second average rate of HTTP requests over the last 5 minutes, useful for monitoring the traffic load on your servers.
rate(http_requests_total[5m])
Gauge Metrics
This instant query fetches the current amount of free memory, providing a snapshot of system resources.
node_memory_MemFree_bytes
Histogram Metrics
The following query calculates the 95th percentile of request latencies over the last 10 minutes, which helps identify outliers in web server performance.
histogram_quantile(0.95, rate(http_request_duration_seconds_bucket[10m]))
Summary Metrics
The query below computes the median latency of processed events, which is crucial for assessing the performance of event-handling systems.
quantile(0.5, rate(processed_events_latency_seconds_sum[5m]) / rate(processed_events_latency_seconds_count[5m]))
Tips for Effective Queries with PromQL:
Use Appropriate Time Ranges: Select time ranges that provide meaningful insights while not overloading the system with long historical data queries.
Leverage Label Filtering: Use labels to filter and refine results, focusing on specific subsets of data.
Optimize for Performance: When writing queries, especially for dashboards or alerts, consider their performance impact and optimize them to run efficiently.
Monitoring the Performance of Milvus Vector Database with Prometheus
Milvus is an open-source, high-performance, and highly scalable vector database that can store, index, and search billion-scale unstructured data through high-dimensional vector embeddings. It is perfect for building modern AI applications such as retrieval augmented generation (RAG), semantic search, multimodal search, and recommendation systems. Milvus runs efficiently across various environments, from laptops and edge devices to large-scale distributed systems.
Prometheus offers comprehensive capabilities to oversee the Milvus vector database's performance. Milvus integrates seamlessly with Prometheus through:
Prometheus Endpoint: Gathers data from various exporters.
Prometheus Operator: Streamlines the management of Prometheus monitoring setups.
Kube-Prometheus: Simplifies full Kubernetes cluster monitoring for robust operation.
Utilizing Prometheus allows you to track critical metrics of Milvus performance such as query response times and resource usage (CPU, GPU, and memory), enabling proactive issue resolution and system optimization. In addition, integrating Prometheus with Grafana further enhances your monitoring framework, providing detailed dashboards for in-depth analysis and efficient maintenance of Milvus deployments tailored to GenAI and similarity search applications.
For comprehensive guidance on setting up Prometheus for Milvus and visualizing metrics with Grafana, explore the resources below:
How to Spot Search Performance Bottleneck in Vector Databases using Prometheus and Grafana
Visualize Milvus Metrics with Grafana | Milvus Documentation
Conclusion
In conclusion, Prometheus is a valuable tool for monitoring various metrics that reflect the health and performance of systems. By using Prometheus's capabilities to track, analyze, and visualize critical operational data, teams can enhance their monitoring practices and make sure that their systems are not only stable but also optimized for efficiency. Whether it's through setting up alerts to catch potential issues early or using detailed dashboards for a clear view of system metrics, Prometheus empowers developers and administrators to maintain high-performance and reliable services.
FAQs
- What are the four types of metrics in Prometheus?
Prometheus categorizes metrics into four types: counters, gauges, histograms, and summaries. Each type serves a specific monitoring purpose, from counting occurrences of events to capturing the distribution of measurements over time.
- How do I choose between using a histogram and a summary?
Choose histograms when you need to capture distributions and are able to define meaningful buckets ahead of time. Use summaries when you need accurate quantile calculations and do not require predefined buckets. The choice depends on your specific use case and performance considerations.
- What is PromQL and how is it used in Prometheus?
PromQL, or Prometheus Query Language, is the powerful language used to query metrics in Prometheus. It allows users to select and aggregate time series data, perform calculations and derive insights from metrics based on specific conditions and time ranges.
- Can I use Prometheus to monitor applications not built in a microservices architecture?
Yes, Prometheus is versatile enough to monitor a wide range of applications, whether they are built using a microservices architecture or a more traditional monolithic approach. It can be configured to scrape metrics from almost any source that exposes data in the Prometheus Exposition Format.
- What are some best practices for labeling and grouping metrics in Prometheus?
When labeling and grouping metrics, ensure that labels are descriptive and consistent across metrics. Avoid high cardinality labels that can degrade performance. Group metrics logically by type or service to enhance clarity and improve query efficiency. This helps maintain an organized monitoring system that is easier to query and manage.
Additional Resources
- What is Prometheus?
- What are the Metrics in Prometheus?
- Metrics Format in Prometheus
- The Four Types of Metrics in Prometheus
- Best Practices for Labeling and Grouping Metrics
- Querying Metrics with PromQL
- Monitoring the Performance of Milvus Vector Database with Prometheus
- Conclusion
- FAQs
- Additional Resources
Content
Start Free, Scale Easily
Try the fully-managed vector database built for your GenAI applications.
Try Zilliz Cloud for Free