APIs Explained: How Apps Talk to Each Other
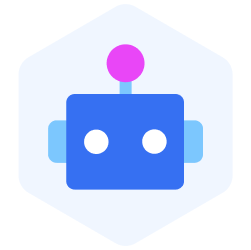
APIs Explained: How Apps Talk to Each Other
What is an API?
An API, or Application Programming Interface, is like a messenger that allows different software applications to talk to each other. Think of it as a bridge that connects two programs to exchange information and work together without having to be the same system.
Technically, an API is a set of rules that defines how one program can request information or services from another program. These rules define:
What information can be requested
How to ask for it (through certain commands)
What format the information should be in when it’s sent back
How Do APIs Work? A Real-World Analogy
A simple way to understand the workings of an API is by comparing it to a real-life scenario at a restaurant illustrated in the following diagram representing the request and response cycle.
The customer places an order (request) through the waiter.
The waiter takes that order to the kitchen (server).
The chef (server) prepares the food (data).
The waiter (API) brings the prepared food (response) back to the customer.
Figure- Real-world Analogy of an API.png
Figure: Real-world Analogy of an API
Customer (User Interacting via Web):The customer represents you or any user interacting with a website or an app. You don’t need to know the details of how the kitchen (server) works. You simply make your request, like choosing food from a menu.
Waiter (API):The waiter is the API. The waiter’s job is to take the customer’s request (such as placing an order) and deliver it to the kitchen. The API, just like the waiter, acts as a messenger between you (the customer) and the server (the chef or kitchen). The API knows exactly how to communicate with the server, much like the waiter knows how to talk to the chef.
Chef (Application Server):The chef in the kitchen is the application server. The chef prepares the meal (processes the request) and hands it back to the waiter (API) who will return the prepared food to the customer. Similarly, the server processes your request and sends the relevant data back to the API.
A practical example is when you use a weather app to check the forecast for your city. The app doesn't generate the weather data itself but rather sends a request to a weather service through an API. The API communicates with the weather service, retrieves the data, and sends it back to your app, where it's displayed in a user-friendly format.
Different Types of APIs
APIs (Application Programming Interfaces) come in several types, categorized by their design, usage, and communication style. Here are the most common types:
1. Web APIs (HTTP APIs)
Web APIs allow communication between servers over the internet using HTTP or HTTPS. They are the most common type of API.
- REST (Representational State Transfer): A popular web API type that uses HTTP methods (GET, POST, PUT, DELETE) and is stateless. REST APIs use URL endpoints to access resources and are widely adopted due to their simplicity and scalability. Common REST API terminology include:
Endpoints: An endpoint is the URL where the API is accessed. It specifies the resource you want to interact with. For example,
https://api.weather.com/forecast
is an endpoint to get weather data.Methods: Methods define the actions you can perform with an API. Common methods include:
GET: Requesting data (e.g., fetching weather information).
POST: Sending data (e.g., submitting a form).
PUT: Updating existing data (e.g., changing user information).
DELETE: Deleting data (e.g., removing an item from a database).
API Key: An API Key is a unique identifier used to authenticate your request to the API. Think of it like a password that proves you're allowed to access certain data or services through the API.
GraphQL: A query language for APIs that allows clients to request exactly the data they need. Unlike REST, where multiple endpoints may be required, GraphQL enables fetching multiple resources in a single request.
SOAP (Simple Object Access Protocol): A protocol-based API that uses XML for message formatting. It’s more rigid and secure than REST and often used in enterprise-level applications requiring high reliability.
JSON-RPC and XML-RPC: Remote procedure call (RPC) protocols that use JSON or XML to encode data. RPC APIs are simpler than REST but are not as flexible or widely adopted.
2. Library-Based APIs
These APIs expose functionalities through programming libraries that developers integrate into their applications. They are not based on network communication but rather on software dependencies.
Language-Specific APIs: Available in specific programming languages (e.g., Python, Java, or JavaScript APIs), these provide pre-built functions to perform tasks within a specific language or framework.
Framework-Specific APIs: APIs designed for specific frameworks, such as Django or Flask for Python, or Spring for Java, help developers build applications faster by abstracting certain functionalities.
3. Operating System APIs
These allow applications to interact with the operating system, providing access to system resources such as file systems, memory, and hardware devices.
Windows API: Allows developers to interact with the Windows OS, handling tasks like file operations, networking, or process management.
POSIX API: Used in Unix-based operating systems, providing standard interfaces for processes, threads, and file management.
4. Database APIs
These APIs allow applications to communicate with databases for performing queries, updating records, and managing transactions.
JDBC (Java Database Connectivity): An API for Java applications to connect to databases and execute SQL queries.
ODBC (Open Database Connectivity): Provides a standard way for applications to interact with databases, regardless of the database management system used.
NoSQL APIs: APIs specific to NoSQL databases like MongoDB, Cassandra, and Elasticsearch, which handle data differently than SQL-based databases.
5. Cloud APIs
Cloud APIs provide access to services in the cloud, allowing applications to leverage resources such as storage, compute, and networking.
AWS SDK/API: Amazon Web Services offers APIs for interacting with its cloud services, such as EC2 (compute), S3 (storage), and Lambda (serverless).
Google Cloud APIs: Provides access to Google Cloud services like BigQuery, Compute Engine, and Kubernetes Engine.
Azure APIs: Microsoft Azure APIs provide services for building and managing applications across cloud-based infrastructure.
6. Hardware APIs
These APIs allow software to communicate directly with hardware components.
DirectX: A collection of APIs for handling tasks related to multimedia, gaming, and video in Microsoft environments.
OpenGL: An API used for rendering 2D and 3D vector graphics, widely used in video games and visualization tools.
Device-Specific APIs: These allow access to hardware features like cameras, sensors, or GPS in mobile or embedded devices (e.g., iOS and Android APIs for mobile hardware).
7. Internal/Private APIs
These APIs are designed for use within an organization and are not exposed to the public. They facilitate communication between internal systems or applications.
- Internal Service APIs: Used for microservices or different components within an organization’s infrastructure to communicate with each other.
8. Public/External APIs
These APIs are exposed to external developers to integrate with third-party services.
Social Media APIs: For accessing features of social platforms like Facebook, Twitter, and LinkedIn (e.g., posting, retrieving data, or managing user profiles).
Payment APIs: Services like PayPal, Stripe, and Square provide APIs for handling transactions, subscriptions, and invoicing.
9. Partner APIs
These APIs are shared with specific business partners, usually under a controlled and limited environment, to enable collaboration and integrations while maintaining privacy and security.
10. Composite APIs
Composite APIs combine multiple APIs into a single call, improving efficiency by reducing the need for multiple requests. They are commonly used in microservice architectures where several services need to be aggregated.
Each type of API serves a specific purpose, and their usage depends on the needs of the application and the environment in which it operates.
Practical Examples of APIs
You probably use APIs every day without even realizing it. Here are some everyday examples:
Logging in with Google or Facebook: Many websites allow you to log in using your Google or Facebook account. When you choose this option, the website uses an API to ask Google or Facebook for your account details (with your permission) so you don’t have to create a new account.
Checking the weather: When you use a weather app, it’s likely using a weather API to fetch the latest forecasts from a weather data provider.
Making payments online: When you make a payment on an e-commerce site, APIs connect the store with payment processors like PayPal or Stripe to complete the transaction securely.
Benefits of APIs
Simplifies Integration
APIs allow systems to work together without requiring developers to rewrite existing code, making it easy to add new features to applications by utilizing external services.
Promotes Reusability
Instead of building everything from scratch, developers can use APIs to integrate commonly used functionalities like payment processing, email services, or user authentication.
Reduces Development Time
APIs speed up development by providing pre-built functionalities that developers can plug into their applications. This reduces the time and effort needed to develop complex features.
Comparison: API vs. SDK
While APIs and SDKs are often mentioned together, they serve different yet complementary roles in software development. Here are some of the main differences between an API and SDK:
Feature | API (Application Programming Interface) | SDK (Software Development Kit) |
Definition | Provides a way for applications to communicate with each other, often over the web. | A set of tools that helps developers create applications tailored to a specific platform. |
Components | Focuses on communication rules, endpoints, and request/response handling. | Includes APIs, development tools, compilers, debuggers, and sometimes hardware simulators. |
Usage | Used to access external services or data, e.g., Google Maps API for location services. | Used to build full applications from scratch, e.g., Android SDK for building Android apps. |
Complexity | Typically lighter and more specific in scope (communication-focused). | Typically more comprehensive, offering a range of tools for application development. |
Example | Twitter API for accessing and posting tweets. | iOS SDK for developing iPhone apps. |
Platform Dependency | Generally platform-independent, especially web APIs. | Typically platform-specific (e.g., iOS SDK for Apple, Android SDK for Android). |
Table: API vs SDK
Milvus/Zilliz APIs: Powering Vector Search and AI Applications
When dealing with large amounts of unstructured data—like images, videos, or audio files—traditional databases are not designed to handle these efficiently. This is where vector databases come into play.
Vector databases such as Milvus and Zilliz Cloud (the managed Milvus) are a type of database management systems that efficiently store, index, and retrieve unstructured data in the form of numerical representations called vector embeddings. They are usually created by embedding models and can capture the semantical relationships of unstructured data.
Milvus ****is an open-source enterprise-level vector database built for performance. It can handle billion-scale vectors with super low latency. It provides various types of APIs catered for different requirements and needs.
RESTful API: Milvus offers a RESTful API that allows developers to manipulate collections and data stored in the vector database. This API provides endpoints for tasks like creating, listing, and querying collections, as well as managing vector data.
Client SDKs: Milvus provides client libraries for various programming languages, including: Python (PyMilvus), Java, Go, C#, and Node.js
These APIs enable developers to perform various operations on Milvus, such as creating collections, inserting data, searching vectors, and managing indexes. The choice of API depends on your specific use case and preferred programming language.
Zilliz Cloud is the fully managed version of the open-source Milvus vector database. It shares the abovementioned RESTful API and Client SDKs Milvus provides. Zilliz Cloud also offers additional groups of APIs for manipulating clusters, collections, and data stored in them.
Control Plane APIs:
Cloud Meta
Cluster Operations
Import Operations
Pipeline Operations
Example Use Case: AI-Powered Image Search
Let’s take a look at an example of using RESTful API to build an AI-powered image search.
Suppose you're building an application where a user uploads an image and finds visually similar images. This involves comparing vector representations of images, where each image is converted into a vector embedding (a numerical representation of its features). Using the RESTful API, you can:
Create collections to store these image vectors.
Insert vectors into the collection.
Query the collection to retrieve similar images based on vector similarity.
The following code demonstrates how to interact with the Zilliz Cloud REST API to manage vector data in a vector database. It includes three key operations: creating a collection to store 128-dimensional image vectors, inserting vectors that represent images, and performing a vector similarity search to find the most similar image based on Euclidean distance.
import requests
import json
# Replace with your Zilliz Cloud API information
API_KEY = 'your_api_key' # Your Zilliz Cloud API Key
ZILLIZ_ENDPOINT = 'https://your-cluster-id.api.your-cloud-region.zillizcloud.com/v1/vector'
HEADERS = {
'Authorization': f'Bearer {API_KEY}',
'Content-Type': 'application/json',
'Accept': 'application/json'
}
# Step 1: Create a collection to store image vectors
def create_image_collection():
url = f"{ZILLIZ_ENDPOINT}/collections/create"
payload = {
"collectionName": "image_collection",
"dimension": 128, # Example vector dimension for images
"metricType": "L2", # Euclidean distance for similarity
"primaryField": "id",
"vectorField": "embedding"
}
response = requests.post(url, headers=HEADERS, json=payload)
print("Create Collection Response:", response.json())
# Step 2: Insert image vectors into the collection
def insert_image_vectors():
url = f"{ZILLIZ_ENDPOINT}/insert"
payload = {
"collectionName": "image_collection",
"data": [
{
"id": 1,
"embedding": [0.12, 0.34, 0.56, 0.78] * 32 # Example 128-dimensional vector
},
{
"id": 2,
"embedding": [0.22, 0.44, 0.66, 0.88] * 32 # Another example vector
}
]
}
response = requests.post(url, headers=HEADERS, json=payload)
print("Insert Image Vectors Response:", response.json())
# Step 3: Search for similar image vectors
def search_similar_images():
url = f"{ZILLIZ_ENDPOINT}/search"
payload = {
"collectionName": "image_collection",
"limit": 1, # Retrieve top 1 similar image
"vector": [0.12, 0.34, 0.56, 0.78] * 32 # Query vector for search
}
response = requests.post(url, headers=HEADERS, json=payload)
print("Search Similar Images Response:", response.json())
# Execute the functions
create_image_collection()
insert_image_vectors()
search_similar_images()
FAQs
1. What is an API in simple terms?
An API (Application Programming Interface) is like a messenger that allows two different applications to communicate with each other. It defines rules and protocols for how one program can request information or services from another, for the purpose of data sharing.
2. How do APIs benefit developers?
APIs save developers time by allowing them to integrate pre-existing functionalities, such as payment processing or social media features, into their apps without building them from scratch. This promotes code reusability, simplifies complex tasks, and speeds up development processes.
3. What’s the difference between an API and an SDK?
An API allows applications to communicate with each other, usually over the web. In contrast, an SDK (Software Development Kit) is a collection of tools, libraries, and documentation that helps developers create applications specifically for a platform, often including APIs as part of the toolkit.
4. What is REST in APIs?
REST (Representational State Transfer) is an architectural style for designing networked applications. REST APIs use HTTP requests to perform operations like getting data (GET), sending data (POST), and updating or deleting data. It's widely used due to its simplicity and flexibility.
5. How is GraphQL different from REST?
GraphQL is a more flexible query language compared to REST. In GraphQL, clients can specify exactly what data they need, which reduces the amount of data transferred. In REST, clients often receive all data from an endpoint, even if only part of it is needed, making GraphQL more efficient in some use cases.
6. What are API keys and why are they important?
API keys are unique identifiers used to authenticate and track API requests. They ensure that only authorized users can access an API and that usage is monitored, which is crucial for controlling access and protecting sensitive data.
7. What is rate limiting in APIs?
Rate limiting is a control mechanism that restricts how many API requests a user can make within a specific time period. This prevents the API server from being overwhelmed by too many requests and ensures fair usage across all users, improving overall performance and reliability.
Related Resources
- What is an API?
- How Do APIs Work? A Real-World Analogy
- Different Types of APIs
- Practical Examples of APIs
- Benefits of APIs
- Comparison: API vs. SDK
- Milvus/Zilliz APIs: Powering Vector Search and AI Applications
- FAQs
- Related Resources
Content
Start Free, Scale Easily
Try the fully-managed vector database built for your GenAI applications.
Try Zilliz Cloud for Free