Unlocking Pre-trained Models: A Developer’s Guide to Audio AI Tasks
Learn how to implement pre-trained models for audio AI applications. Explore speech recognition, audio classification, and TTS with practical code examples.
Read the entire series
- Top 10 Most Used Embedding Models for Audio Data
- Unlocking Pre-trained Models: A Developer’s Guide to Audio AI Tasks
- Choosing the Right Audio Transformer: An In-depth Comparison
- From Text to Speech: A Deep Dive into TTS Technologies
- Getting Started with Audio Data: Processing Techniques and Key Challenges
- Enhancing Multimodal AI: Bridging Audio, Text, and Vector Search
- Scaling Audio Similarity Search with Vector Databases
Introduction
Artificial intelligence has revolutionized audio processing, enabling applications like speech recognition, music analysis, and audio classification. These advancements have transformed industries (e.g., entertainment, customer service), making it easier to interact with machines using voice commands, analyze vast amounts of audio data, and even generate realistic synthetic speech. However, training deep learning models from scratch can be resource-intensive, requiring vast amounts of labeled data and substantial computational power.
Pre-trained models provide a powerful shortcut, enabling developers to use state-of-the-art AI without requiring vast training data or high computational costs. These models, trained on large-scale datasets, capture essential patterns and can be adapted for specific applications with minimal effort. Using pre-trained models, developers can build sophisticated audio AI applications faster, more efficiently, and with significantly lower costs.
This blog explores the significance of pre-trained models in audio AI, their applications, and how developers can integrate them into their projects. From speech recognition to speech-to-text synthesis, we’ll analyze the best models available and the tools that simplify their implementation.
Pre-trained Models: Building Blocks for Audio AI
What Are Pre-trained Models?
Pre-trained models are neural networks already trained on large datasets for specific tasks. These models learn complex patterns from data, providing developers with a strong foundation for their applications. Instead of training a model from scratch, developers can fine-tune or directly use pre-trained models to perform speech recognition, text-to-speech, and sound classification tasks. They leverage massive computing resources and datasets often unavailable to individual developers, making cutting-edge technology accessible with minimal effort.
The models can be developed using different training approaches, including supervised, semi-supervised, and self-supervised learning methods. Supervised models rely on labeled datasets, requiring human-annotated examples for training. In contrast, self-supervised models, such as Wav2Vec 2.0, learn directly from raw, unlabeled audio by predicting missing or masked parts of the input. This enables them to extract meaningful representations without extensive manual labeling, making them especially valuable for real-world applications where labeled data is scarce or expensive to obtain.
Why Developers Should Care
Pre-trained models save time, reduce computational costs, and enable rapid prototyping. They provide a strong foundation for developers looking to build high-performance audio AI applications without requiring deep expertise in deep learning. Optimized for efficiency, these models are suitable for deployment on edge devices and cloud environments.
Another key advantage is their continuous improvement, with newer versions often outperforming previous iterations. Developers can benefit from ongoing improvements without having to retrain models from scratch. Additionally, pre-trained models lower the barrier to entry for smaller organizations, enabling them to deploy AI-driven audio applications without needing extensive in-house AI expertise.
Relevance to Audio AI Use Cases
As audio AI continues to evolve, its applications are becoming increasingly diverse. From virtual assistants that understand natural speech to automated music analysis and industrial monitoring systems, audio AI is reshaping the way machines interact with sound. Audio AI spans multiple domains, including:
Speech recognition: Converting spoken language into text.
Audio classification: Identifying sounds in an environment.
Text-to-speech (TTS): Synthesizing natural-sounding speech from text.
Music analysis: Recognizing patterns in music, such as genre classification.
Speaker identification: Determining who is speaking in an audio recording.
Audio anomaly detection: Identifying unusual sounds in industrial applications, such as detecting machine failures.
Environmental sound recognition: Detecting background noises for applications like smart home automation.
Pre-trained models have significantly accelerated advancements in these areas by eliminating the need for large-scale labeled datasets and extensive training from scratch. For example, models like Whisper and DeepSpeech have made speech recognition more accessible, while Tacotron and WaveNet have revolutionized text-to-speech synthesis. These advancements have not only improved accuracy but also enhanced real-time processing, making AI-powered audio applications increasingly practical and effective for everyday use.
Popular Audio AI Tasks and Suitable Pre-trained Models
Several pre-trained models cater to different audio AI applications, from which the most popular are the following:
Speech Recognition
Whisper (by OpenAI): A highly accurate automatic speech recognition (ASR) model capable of multilingual transcription. It has been trained on diverse datasets, making it robust across various accents and languages.
Wav2Vec 2.0 (by Meta AI): A self-supervised model that learns representations from raw audio, useful for speech-to-text tasks. It performs well with limited labeled data and can be fine-tuned for specialized applications.
Audio Classification
YAMNet: Identifies sound events from everyday environments, such as sirens, music, and speech. It is useful for applications requiring real-time sound recognition, such as smart assistants.
OpenL3: Extracts audio embeddings for music and environmental sound analysis. It can be integrated with machine learning pipelines to enhance classification performance in niche areas.
Text-to-Speech (TTS)
Tacotron: Generates natural-sounding speech with deep learning-based speech synthesis. It uses sequence-to-sequence modeling to generate high-quality, human-like speech.
VALL-E (by Microsoft): A powerful TTS model that can generate realistic speech from minimal training data. It excels in voice cloning, where it can replicate a speaker's tone and style with just a few seconds of reference audio
Each of these audio AI categories—speech recognition, audio classification, and text-to-speech—offers unique capabilities that can be adapted for a wide range of applications. Speech recognition models like Whisper and Wav2Vec 2.0 focus on accurately transcribing spoken language into text, serving a variety of tasks from transcription to real-time communication. Audio classification models like YAMNet and OpenL3 enable machines to identify and categorize sounds in environments, making them ideal for applications such as smart assistants or environmental monitoring. Finally, text-to-speech models like Tacotron and VALL-E excel at generating natural-sounding speech, with the added benefit of enabling features like voice cloning and creating lifelike synthetic speech. Together, these models form the backbone of many AI-driven audio applications, making them accessible, scalable, and highly versatile.
Benefits of Pre-trained Models for Developers
Pre-trained models offer significant benefits, such as reducing development time, lowering costs, and improving the quality of AI applications. By using pre-trained models, developers can enhance their applications in the following ways:
Faster development cycles: Skip the costly process of training models from scratch.
High performance: Leverage state-of-the-art models trained on vast datasets.
Lower computational costs: Use optimized models that can run efficiently on various hardware.
Flexibility: Fine-tune models for domain-specific tasks or use them as-is for general applications.
Lower data requirements: Many pre-trained models perform well even with limited labeled data, making them suitable for applications where data collection is expensive or difficult.
These models not only save time and reduce costs but also enable teams to focus on creating innovative solutions. Whether for voice assistants, real-time audio classification, or personalized speech synthesis, the versatility of pre-trained models empowers developers to push the boundaries of audio applications development..
How to Work with Pre-trained Audio Models
1. Use Hugging Face Pipelines for Quick Deployment
Hugging Face provides easy-to-use pipelines for deploying pre-trained audio models with minimal code. For instance, running speech recognition using Wav2Vec 2.0 can be done with just a few lines of code:
from transformers import pipeline
# Initialize the ASR pipeline with Wav2Vec 2.0
asr_pipeline = pipeline("automatic-speech-recognition",
model="facebook/wav2vec2-large-960h")
# Transcribe audio file
result = asr_pipeline("path_to_audio.wav")
# Print the transcription
print(result["text"])
This short code snippet sets up a speech recognition pipeline that takes an audio file and returns the transcribed text. Additionally, Hugging Face supports quick fine-tuning of pre-trained models on custom datasets, which is shown in the following example.
2. Fine-Tune for Domain-Specific Tasks
While pre-trained models work well in general scenarios, fine-tuning on domain-specific datasets improves accuracy for niche applications. Fine-tuning involves retraining the model on smaller datasets that are relevant to the target application, allowing it to better recognize domain-specific terminology and speech patterns.
For instance, let’s fine-tune the Wav2Vec 2.0 model on the Speech Commands dataset, which contains short, one-second .wav files, each with a spoken word or background noise. This dataset is commonly used for simple classification tasks and includes a variety of speakers.
Step 1: Load the Dataset
By loading a small subset for training, you can quickly experiment with fine-tuning the model while saving computational resources.
import torch
from transformers import Wav2Vec2ForSequenceClassification, Wav2Vec2Processor
from datasets import load_dataset
from transformers import Trainer, TrainingArguments
import librosa
# Load Speech Commands dataset
dataset = load_dataset("speech_commands", 'v0.01', split="train[:3%]")
Step 2: Load the Model
In this step, you initialize the pre-trained Wav2Vec 2.0 model and processor. The processor handles the conversion of raw audio into input features that can be understood by the model, while the model itself is ready for sequence classification tasks.
# Initialize Wav2Vec2 model and processor for sequence classification
processor = Wav2Vec2Processor.from_pretrained("facebook/wav2vec2-base-960h")
model = Wav2Vec2ForSequenceClassification.from_pretrained("facebook/wav2vec2-base-960h", num_labels=4)
Step 3: Preprocess the Dataset
Preprocessing involves transforming the audio data into a format suitable for input into the model. This includes resampling the audio to a consistent sample rate and padding the sequences to ensure they are uniform in length, which improves training performance.
# Preprocessing function
def preprocess_audio(example):
# Get the audio array
audio_array = example["audio"]["array"]
original_sr = example["audio"]["sampling_rate"]
# Resample the audio to 16kHz
audio_resampled = librosa.resample(audio_array, orig_sr=original_sr, target_sr=16000)
# Process the audio with Wav2Vec2 processor and pad the sequences
example["input_values"] = processor(audio_resampled, sampling_rate=16000, return_tensors="pt", padding="max_length", max_length=16000).input_values[0]
# Remap the labels to a range of 0 to 1
label_mapping = {20: 0, 21: 1}
example["label"] = label_mapping.get(example["label"], -1)
return example
# Apply the preprocessing function
preprocessed_dataset = dataset.map(preprocess_audio, remove_columns=["audio", "file", "is_unknown", "speaker_id", "utterance_id"])
Step 4: Perform Train/Test Split
Splitting the dataset ensures that the model is trained on one portion (train dataset) and evaluated on another (test dataset). Stratified splitting helps maintain the same distribution of labels across both datasets, which is particularly important when the data is imbalanced.
import pandas as pd
from sklearn.model_selection import train_test_split
from datasets import Dataset
# Convert to pandas DataFrame
df = pd.DataFrame(preprocessed_dataset)
# Perform stratified splitting using the "label" column
train_df, eval_df = train_test_split(df, test_size=0.2, stratify=df["label"])
# Convert back to HuggingFace dataset format
train_dataset = Dataset.from_pandas(train_df)
eval_dataset = Dataset.from_pandas(eval_df)
Step 5: Fine Tune the Model
During fine-tuning, the model learns to adapt to the new dataset by adjusting its internal parameters. The training and evaluation metrics provide insight into how well the model is performing on both the training data and the unseen test data.
# Define training arguments
training_args = TrainingArguments(
output_dir="./results",
per_device_train_batch_size=1,
eval_strategy="epoch",
num_train_epochs=3,
logging_steps=10,
save_steps=500,
save_total_limit=2,
remove_unused_columns=False,
report_to="none",
no_cuda=False,
dataloader_pin_memory=False
)
# Define the Trainer
trainer = Trainer(
model=model.to('cuda'),
args=training_args,
train_dataset=train_dataset,
eval_dataset=eval_dataset,
data_collator=lambda data: {
"input_values": torch.stack([torch.tensor(x["input_values"]) for x in data]).to('cuda'),
"labels": torch.tensor([x["label"] for x in data]).to('cuda')
},
processing_class=processor,
)
# Train the model
trainer.train()
# Evaluate the model
results = trainer.evaluate()
print(results)
Output:
{'eval_loss': 0.6443880796432495,
'eval_runtime': 4.0858,
'eval_samples_per_second': 75.139,
'eval_steps_per_second': 9.545,
'epoch': 3.0}
3. Generate Embeddings for Vector Search Applications
Vector search enables efficient retrieval of similar data points using embeddings—numerical representations of content. For audio data, embeddings can be generated directly from the raw audio file or from its transcription. This flexibility allows for different types of audio search applications, such as speaker recognition and semantic text-based search. Below are two approaches for generating embeddings using Wav2Vec 2.0 for the audio embedding and Whisper for the transcription embedding, followed by storing and querying it in the Milvus vector database.
Generate Embeddings from Audio File
This approach extracts embeddings directly from the raw audio waveform, preserving audio-specific features such as tone and speaker characteristics.
import torch
from transformers import Wav2Vec2FeatureExtractor, Wav2Vec2Model
import librosa
import numpy as np
# Load pre-trained Wav2Vec 2.0 model and feature extractor
feature_extractor = Wav2Vec2FeatureExtractor.from_pretrained("facebook/wav2vec2-base")
model = Wav2Vec2Model.from_pretrained("facebook/wav2vec2-base")
def generate_audio_embedding(audio_path):
# Load audio file
audio_input, sample_rate = librosa.load(audio_path, sr=16000)
# Preprocess the audio
input_values = feature_extractor(audio_input, sampling_rate=sample_rate, return_tensors="pt").input_values
# Generate embedding
with torch.no_grad():
outputs = model(input_values)
# Use the last hidden state as the embedding
embedding = outputs.last_hidden_state.mean(dim=1).squeeze().numpy()
return embedding
# Example usage
audio_file_path = '/content/male.wav'
audio_embedding = generate_audio_embedding(audio_file_path)
# Print embedding details
print("Embedding shape:", audio_embedding.shape)
print("First few embedding values:", audio_embedding[:5])
Output:
Embedding shape: (768,)
First few embedding values: [-0.03371317 -0.05751501 0.18964688 0.10422201 0.16818957]
Generate Embeddings from Audio Transcription
Instead of using raw audio, this approach first transcribes the speech using Whisper and then generates embeddings from the transcribed text. This is useful for text-based semantic searches.
import openai
import whisper
import numpy as np
import os
from langchain_milvus import Milvus
from langchain_openai import OpenAIEmbeddings
from langchain_core.documents import Document
from google.colab import userdata
# Step 1: Load Whisper model
model = whisper.load_model("base.en")
# Step 2: Transcribe audio using Whisper
audio_file = '/content/male.wav'
result = model.transcribe(audio_file)
transcribed_text = result["text"]
# Step 3: Generate OpenAI embeddings for the transcribed text
os.environ["OPENAI_API_KEY"] = userdata.get('OPENAI_API_KEY')
# Step 4: Set up Milvus vector store with OpenAI embeddings
URI = "./milvus_example.db" # Local URI for Milvus Lite or use
embedding_function = OpenAIEmbeddings(model="text-embedding-ada-002")
vector_store = Milvus(
embedding_function=embedding_function,
connection_args={"uri": URI},
index_params={"index_type": "FLAT", "metric_type": "L2"},
auto_id=True
)
# Step 5: Wrap the transcribed text in a Document and add to Milvus
doc = Document(page_content=transcribed_text)
vector_store.add_documents(documents=[doc])
# Step 6: Perform a similarity search using Milvus (querying the same audio)
similar_results = vector_store.similarity_search_with_relevance_scores(transcribed_text, k=1)
# Step 7: Print results
print("Similarity Score:", similar_results[0][1])
Output:
Similarity Score: 1.0
A high similarity score indicates that the query text closely matches stored transcriptions, making this approach ideal for text-based retrieval tasks.
Tools and Frameworks for Pre-trained Audio Models
To work effectively with pre-trained audio models, developers can leverage a variety of tools and frameworks that simplify deployment, fine-tuning, and feature extraction. These tools provide essential functionalities such as speech recognition, text-to-speech (TTS), and audio embedding generation.
Here are some key tools used in the field:
Hugging Face Transformers: Offers pre-trained models for speech recognition, text-to-speech, and audio classification, making it easy to integrate powerful AI capabilities into applications.
PyTorch & TensorFlow: These deep learning frameworks provide the flexibility to train, fine-tune, and deploy models efficiently, with support for GPU acceleration.
Milvus: Essential for vector search applications, these tools enable fast retrieval of similar audio samples based on embeddings.
Librosa & torchaudio: Provide advanced audio processing functionalities, such as feature extraction, resampling, and spectrogram generation.
DeepSpeech: An open-source ASR engine developed by Mozilla that offers on-device speech recognition capabilities, making it useful for offline applications.
ESPnet: A flexible end-to-end speech processing toolkit supporting automatic speech recognition (ASR), text-to-speech (TTS), and speech translation.
Each of these tools plays a crucial role in enhancing the capabilities of pre-trained models, allowing developers to tailor solutions for real-world speech applications. Depending on the use case, whether it’s real-time speech transcription, speaker verification, or voice synthesi, choosing the right framework can significantly improve performance and efficiency.
Challenges When Using Pre-trained Models
Despite their numerous advantages, pre-trained audio models also come with challenges that can impact performance, usability, and deployment. Some of the key challenges include:
Domain Mismatch: Many pre-trained models are trained on general datasets, such as English news or podcast transcripts. When applied to domain-specific audio (e.g., medical dictation, legal recordings, or accented speech), performance may degrade. Fine-tuning on domain-relevant datasets is often necessary to bridge this gap.
Noisy Audio Limitations: Background noise, reverberation, and poor-quality recordings can significantly reduce the accuracy of speech recognition models. While some pre-trained models include noise-robust features, additional denoising techniques or custom model training may be required for optimal results.
Dataset Constraints: Certain languages, dialects, and low-resource speech datasets are underrepresented in pre-trained models. This can limit accuracy for multilingual or regional applications. In such cases, transfer learning or data augmentation may be needed to improve performance.
Computational Resources: Although pre-trained models reduce training time, deploying large models may require high-performance GPUs or cloud-based infrastructure. Optimizations such as quantization and model pruning can help make deployments more efficient.
To overcome these challenges, developers must carefully evaluate the model’s strengths and weaknesses in their target domain.
Conclusion
Pre-trained models provide a game-changing advantage for developers working on Audio AI applications. They offer a fast, efficient, and cost-effective way to implement complex audio tasks without starting from scratch. By leveraging these models, developers can focus on refining applications and improving performance rather than investing time and resources into model training.
As the field of AI continues to evolve, we can expect even more advancements in pre-trained models, leading to improved accuracy, efficiency, and accessibility. Developers should explore different models, experiment with fine-tuning techniques, and integrate them into real-world applications to unlock their full potential. Whether you are building a speech recognition system, an audio classifier, or a text-to-speech engine, pre-trained models serve as a valuable tool to accelerate your progress.
By staying informed about the latest trends in Audio AI and adopting cutting-edge tools, developers can stay ahead of the curve and build innovative, high-performing solutions.
Stay tuned for our next deep dive: “From Text to Speech: A Deep Dive into TTS Technologies.”
- Introduction
- Pre-trained Models: Building Blocks for Audio AI
- Popular Audio AI Tasks and Suitable Pre-trained Models
- How to Work with Pre-trained Audio Models
- Tools and Frameworks for Pre-trained Audio Models
- Challenges When Using Pre-trained Models
- Conclusion
Content
Start Free, Scale Easily
Try the fully-managed vector database built for your GenAI applications.
Try Zilliz Cloud for FreeKeep Reading
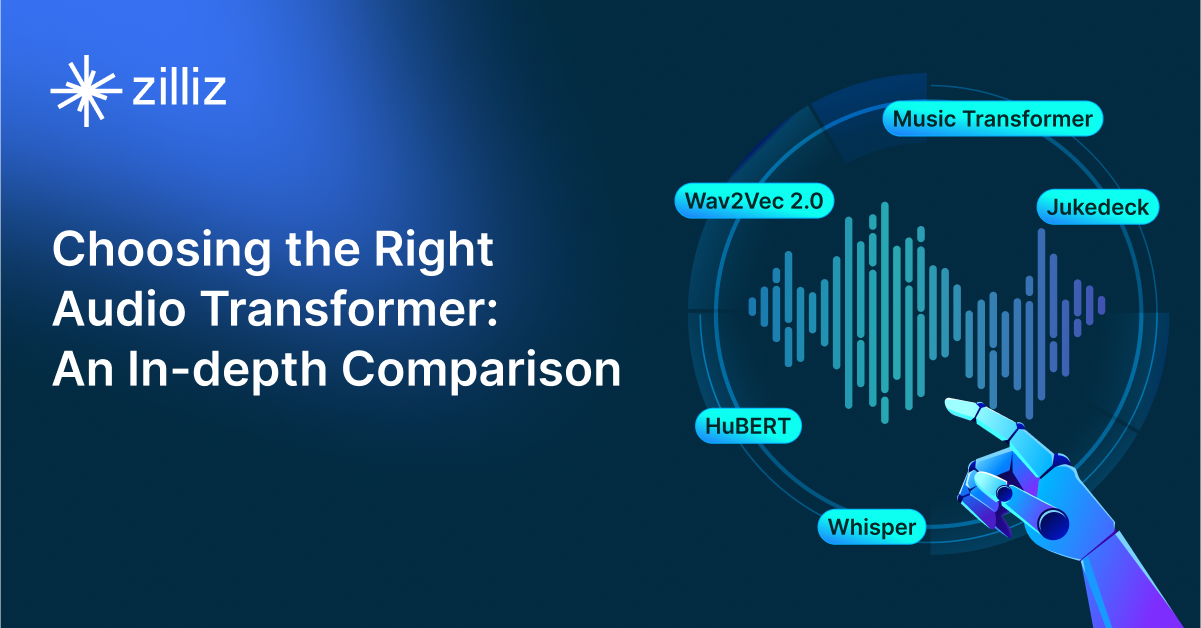
Choosing the Right Audio Transformer: An In-depth Comparison
Discover how audio transformers enhance sound processing. Explore their principles, selection criteria, popular models, applications, and key challenges.
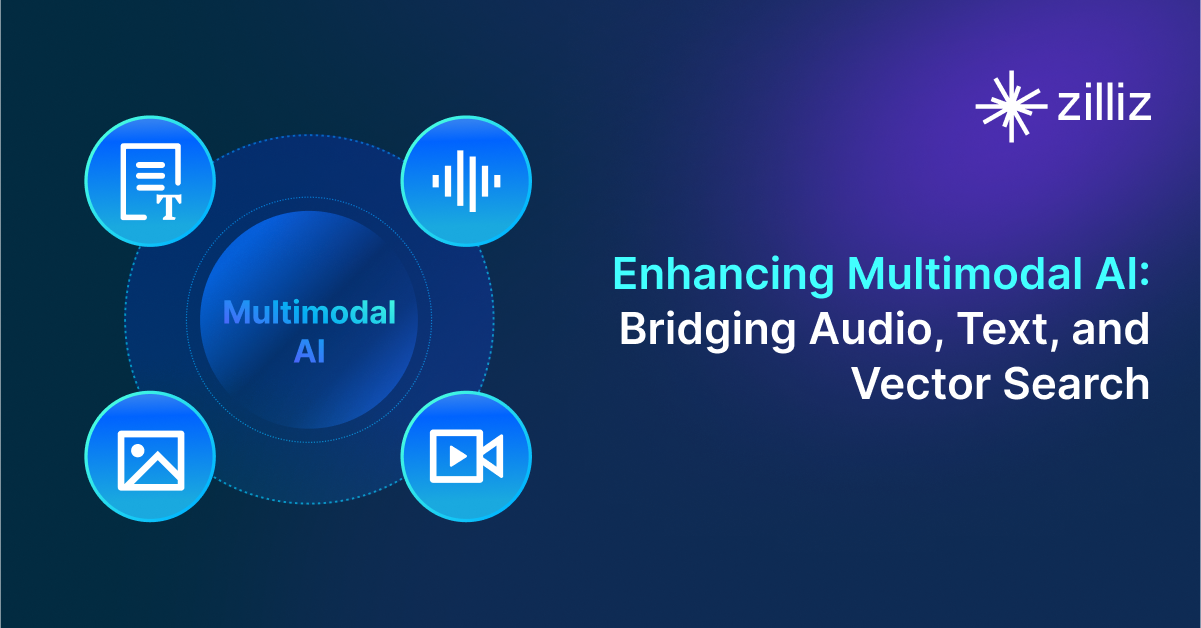
Enhancing Multimodal AI: Bridging Audio, Text, and Vector Search
In this article, we will explore how multimodal AI enhances AI systems by bridging audio, text, and vector search.
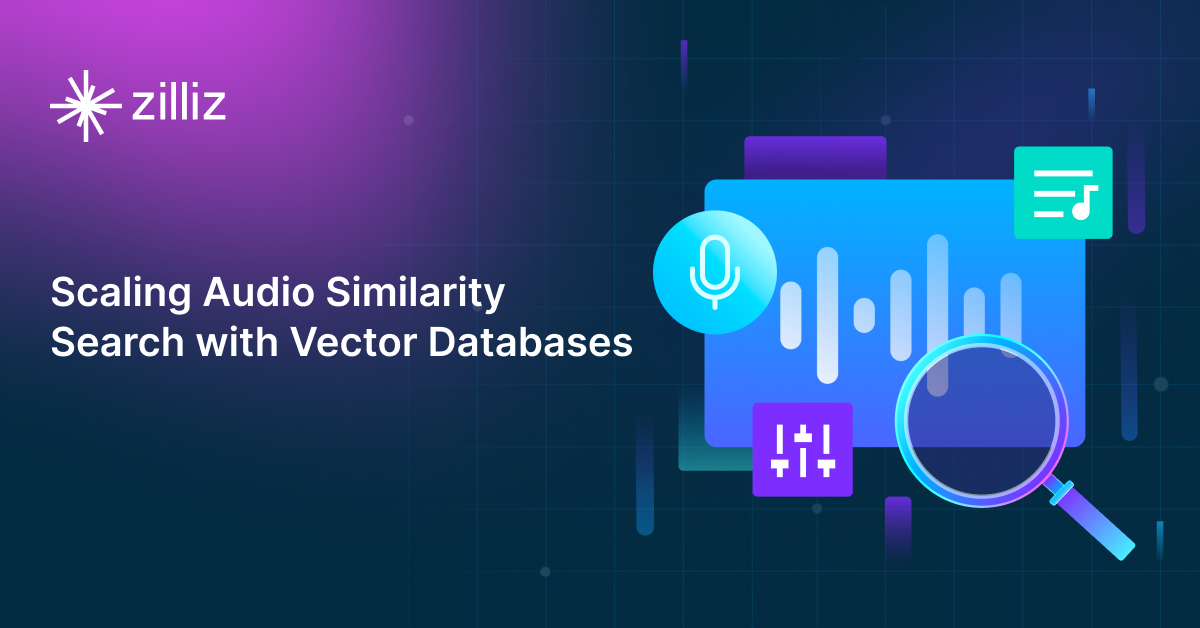
Scaling Audio Similarity Search with Vector Databases
Discover how vector databases like Milvus and Zilliz Cloud enable efficient audio similarity search at scale, transforming music recommendations and audio retrieval applications.