Beyond Basic RNNs: A Practical Guide to Gated Recurrent Units
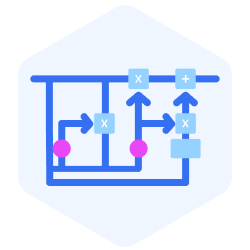
Beyond Basic RNNs: A Practical Guide to Gated Recurrent Units
Gated Recurrent Units (GRUs) are a simpler Recurrent Neural Network (RNN) type that handles sequential data like text or time series. They use small “gates” to decide what information to keep or discard at each step, which helps them remember long-term patterns and avoid the vanishing gradient problem in older Recurrent Neural Networks (RNNs). Because GRUs have fewer parameters than Long Short-Term Memory (LSTM), they tend to train faster without sacrificing accuracy. This makes them a popular choice in tasks such as Natural Language Processing (NLP), speech recognition, and forecasting. By balancing simplicity and performance, GRUs have become a go-to solution for many real-world applications that rely on sequence data.
Background: From RNNs to GRUs
Limitations of Traditional RNNs
Traditional RNNs process a sequence by passing information from one time step to the next. They take an input (like a single word in a sentence) and combine it with the hidden state from the previous step. This repeated process, however, leads to significant issues like:
No Gating Mechanism: Standard RNNs lack a structured way to decide what past information is essential and what should be forgotten. They simply combine new inputs with the old hidden state, which can lead to outdated or irrelevant details lingering in the network.
Vanishing Gradients: As sequences lengthen, the gradients updating the weights become extremely small. The network struggles to learn long-term patterns because those small gradients barely adjust the parameters.
Exploding Gradients: In some cases, gradients can grow too large, causing the training process to become unstable. This usually results in the model producing meaningless predictions or “blowing up” during training.
Inefficient Memory Management: Without gates, the network cannot selectively filter out unhelpful past information. This one-size-fits-all approach can cause the memory of previous time steps to become cluttered with data that doesn’t contribute to the current output.
How do GRUs Solve the Limitations of Traditional RNN?
- Gated Mechanisms
Unlike standard RNNs, GRUs use two main gates: the Update and Reset gates. These gates act like filters that control the flow of information at each time step. This structure gives the model a more direct way to manage how much past data it should carry forward.
- Improved Memory Management
Reset Gate: Decides how much of the old hidden state to clear out if it’s no longer relevant.
Update Gate: Balances old and new information, helping the model retain only what truly matters. This targeted control means the network can remember significant details over extended time spans and discard anything that’s not useful.
- Mitigating Vanishing Gradients
GRUs address these limitations by introducing gates that control how information flows through the network. Instead of relying on a single hidden state update at every step, GRUs use specialized mechanisms to decide how much past information to keep or discard. This design helps maintain necessary signals over long sequences, thus reducing the risk of vanishing gradients. It also keeps the network stable during training by preventing gradients from growing out of control.
- Faster Training
GRUs often train faster than standard RNNs and require fewer training epochs to perform well. By focusing on what matters most at each time step, the network uses its resources more efficiently. Hence, it’s a strong choice for tasks that involve long sequences.
How Does GRU Work?
A GRU cell uses an update gate and a reset gate to manage what information gets passed along as the network processes a sequence. This gating mechanism helps the network remember essential details for longer and avoid common RNN issues like vanishing gradients. At each time step, the cell decides:
How much of the old, hidden state should be kept?
How much of the old, hidden state to forget.
How to combine the new input with the retained information.
To understand how GRUs process information, let’s break it down step by step:
Figure: Architecture of GRU
1. Input and Previous Hidden State
At each time step, a GRU takes in two key inputs:
Current Input Vector (xₜ): The data at the present time step.
Previous Hidden State (hₜ₋₁): The memory from the last step, which helps maintain context over time.
These two inputs pass through the GRU cell, where a series of operations update the hidden state for the next time step.
2. Reset Gate (rₜ)
The reset gate determines how much of the previous hidden state should be forgotten before incorporating new input. It operates as follows:
If rₜ is close to 0, the GRU discards most of the past information, allowing the model to focus on recent inputs.
If rₜ is close to 1, the GRU retains previous knowledge, preserving historical context.
This functionality is useful when dealing with sequences where older information may or may not be relevant to the current step.
3. Candidate Hidden State (h̃ₜ)
Once the reset gate has adjusted the memory, the GRU computes a candidate hidden state. This potential new memory combines the modified past state with the current input. The candidate hidden state is usually passed through a tanh activation function, which helps capture complex, non-linear patterns in the data.
4. Update Gate (zₜ)
The update gate determines how much of the old hidden state should be carried forward versus how much should be replaced with new information. Its behavior can be summarized as follows:
If zₜ is close to 1, the GRU prioritizes fresh information, making it highly responsive to new inputs.
If zₜ is close to 0, the GRU retains past knowledge, maintaining long-term dependencies.
This gate is essential for preventing unnecessary overwriting of important information from earlier time steps.
5. Final Hidden State (hₜ)
The final output of the GRU for the current time step is a weighted combination of the previous hidden state (hₜ₋₁) and the candidate hidden state (h̃ₜ). The update gate (zₜ) determines this balance:
hₜ = (1 - zₜ) * hₜ₋₁ + zₜ * h̃ₜ
By dynamically controlling this balance, the GRU ensures that it retains crucial information while adapting to new inputs. This capability makes GRUs effective in applications such as speech recognition, language modeling, and time-series forecasting.
GRU vs. LSTM: Key Differences
RNNs struggled with vanishing gradients, making it difficult to learn long-term dependencies. To address this, Long Short-Term Memory (LSTMs) and GRUs introduced gating mechanisms to regulate information flow through time steps. Both architectures improve memory retention, but they differ in structure, complexity, and efficiency.
While both GRUs and LSTMs are widely used for sequential data tasks, choosing between them depends on factors like training speed, memory efficiency, and task complexity. Below is a detailed comparison of their main aspects:
Aspect | GRU | LSTM |
Number of Gates | 2 (Update, Reset) | 3 (Input, Forget, Output) |
Parameter Count | Typically fewer (due to fewer gates) | Generally more parameters |
Training Speed | Often faster because of fewer parameters | Can be slower with larger models |
Memory Usage | Lower, making it more efficient in some cases | Higher, which might be a constraint in resource-limited setups |
Performance | Matches or exceeds LSTMs in many tasks | Often does equally well, especially with complex sequences |
Gate Mechanism Complexity | Simpler gating mechanism | More complex but can capture subtle dependencies |
Recommended Use Cases | Tasks needing faster training or fewer resources | Tasks where extremely long sequences or complex dependencies exist |
Table: GRU vs LSTM
Implementation in Python
Below is a simple example of building and training a GRU-based model using PyTorch. This code shows the basic setup, including defining a GRU class, training on dummy data, and printing the loss at each epoch.
The code is also available on Kaggle as a Notebook. You can adapt these ideas to suit your own dataset and tasks.
Setting Up the Environment
Make sure you have PyTorch installed. You can install it with:
pip install torch torchvision torchaudio
Complete Code Example
import torch
import torch.nn as nn
import torch.optim as optim
# Define the GRU-based model
class GRUModel(nn.Module):
def __init__(self, input_size, hidden_size, num_layers, output_size):
super(GRUModel, self).__init__()
self.hidden_size = hidden_size
self.num_layers = num_layers
# batch_first=True means the input shape is (batch, seq_len, input_size)
self.gru = nn.GRU(input_size, hidden_size, num_layers, batch_first=True)
self.fc = nn.Linear(hidden_size, output_size)
def forward(self, x):
# Initialize hidden states to zeros
h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size)
# Forward pass through the GRU
out, _ = self.gru(x, h0)
# We take the output from the last time step and pass it through a fully connected layer
out = self.fc(out[:, -1, :])
return out
# Hyperparameters
input_size = 10 # Number of features in each input step
hidden_size = 16 # Number of features in the hidden state
num_layers = 1 # Number of GRU layers
output_size = 1 # Target dimension (e.g., regression)
learning_rate = 0.001
num_epochs = 10
# Create the model, define loss and optimizer
model = GRUModel(input_size, hidden_size, num_layers, output_size)
criterion = nn.MSELoss()
optimizer = optim.Adam(model.parameters(), lr=learning_rate)
# Generate some dummy data for demonstration
# Suppose we have a sequence length of 5, and each element in the sequence has 10 features
X_train = torch.randn(100, 5, input_size) # 100 samples, each is a sequence of length 5
y_train = torch.randn(100, output_size) # 100 target values
# Training loop
for epoch in range(num_epochs):
model.train()
# Reset gradients
optimizer.zero_grad()
# Forward pass
outputs = model(X_train)
# Calculate the loss
loss = criterion(outputs, y_train)
# Backward pass (compute gradients)
loss.backward()
# Update parameters
optimizer.step()
print(f"Epoch [{epoch+1}/{num_epochs}], Loss: {loss.item():.4f}")
Output
Epoch [1/10], Loss: 0.9914
Epoch [2/10], Loss: 0.9868
Epoch [3/10], Loss: 0.9823
Epoch [4/10], Loss: 0.9778
Epoch [5/10], Loss: 0.9734
Epoch [6/10], Loss: 0.9691
Epoch [7/10], Loss: 0.9648
Epoch [8/10], Loss: 0.9606
Epoch [9/10], Loss: 0.9564
Epoch [10/10], Loss: 0.9523
Code Explanation
Model Architecture:
- The
GRUModel
class uses a single GRU layer (nn.GRU
) withbatch_first=True
, meaning the input is expected in the format(batch_size, sequence_length, input_size)
. - The final
nn.Linear
layer converts the last time step’s hidden output to the desiredoutput_size
, which could be a single value (e.g., for regression) or multiple classes.
Hidden State Initialization:
We create a zero-initialized hidden state
h0
inside theforward
method. For some tasks, you may need to fine-tune this initialization or move it outside to handle multiple batches differently.Training Loop:
- In each epoch, we reset gradients, run a forward pass, compute the loss (
MSELoss
in this example), and then backpropagate and update the model parameters withoptimizer.step()
.
- In each epoch, we reset gradients, run a forward pass, compute the loss (
Tips and Best Practices
- Use GPUs: If you have a GPU available, you can move your tensors and model to the GPU for faster training by calling
X_train = X_train.cuda()
, etc. - Tune Hyperparameters: Adjust
hidden_size
,num_layers
,learning_rate
, andnum_epochs
based on your dataset and specific task. - Real Data: Replace the dummy data with your own dataset in the shape (
batch_size
,sequence_length
,input_features
). - Model Complexity: Add more layers or adjust the hidden size if your data requires a deeper or more expressive model.
Use Cases & Applications
Natural Language Processing (NLP): GRUs capture context over multiple words or sentences in machine translation (e.g., translating English to French), sentiment analysis (understanding the tone of social media posts), and text classification (categorizing emails or news articles).
Time-Series Forecasting: GRUs model patterns in sequential data, such as stock prices, weather conditions, or energy usage. By learning trends and seasonality in historical data, they can more accurately predict future values, which is crucial in finance, climate monitoring, and industrial IoT.
Speech Processing: GRUs are used in end-to-end speech recognition systems to process audio signals over time to convert spoken language into text. They’re also useful for audio generation or noise reduction by recognizing and preserving main acoustic features.
Recommendation Systems: These networks learn from a user’s interaction history—like clicks, views, or purchases—to suggest relevant products or content. GRUs handle sessions of varying length and adapt quickly to changes in user preferences.
Healthcare Diagnostics: GRUs analyze time-stamped medical data, such as patient vitals or electrocardiogram (ECG) signals, to predict health outcomes. They can help detect early signs of heart irregularities or identify patients at risk of readmission.
Anomaly Detection: GRUs learn normal behavioral patterns in systems like network traffic or manufacturing pipelines. When real-time data deviates from these norms, they can promptly flag potential security breaches or mechanical failures.
Advantages of GRU
Mitigates Vanishing Gradients: The gating architecture allows important information to flow more efficiently, thus reducing the risk that gradients shrink to near zero over long sequences.
Fewer Parameters vs. LSTM: Because GRUs have only two gates instead of three, models typically have fewer trainable parameters, which can lead to faster training and easier tuning.
Practical Performance: GRUs perform well on tasks like language modeling, time-series forecasting, and recommendation systems, often matching or surpassing more complex architectures.
Faster Convergence: By focusing on relevant information at each time step, GRUs can converge more quickly during training, saving time and computational resources.
Limitations of GRU
Computationally Intensive for Very Long Sequences: Although GRUs handle moderate sequence lengths well, extremely long sequences may still cause high computational costs.
Hyperparameter Sensitivity: Choosing the right hidden size, number of layers, and learning rate can significantly impact results and may require extensive experimentation.
Limited Applicability in Certain Domains: While GRUs are generally versatile, specialized architectures might outperform them in tasks with highly structured data, such as certain computer vision problems or graph-related tasks.
Empowering GRUs with Milvus: The Perfect Match for Vector Search
Training a GRU model gives you powerful representations of sequential data, whether text, time-series signals, or user behavior patterns. But once you have these vector embeddings, where do you store and query them? That’s where Milvus (created by Zilliz engineers) comes in. As a vector database, Milvus can efficiently manage large volumes of high-dimensional embeddings to perform fast similarity searches, clustering, and more.
Why Store GRU Embeddings in Milvus?
Storing embeddings generated by a GRU model in Milvus unlocks powerful vector-based search and analysis. Below are main reasons to pair these two technologies, along with real-world examples illustrating their value.
- Instant Similarity Searches
Milvus indexes embeddings in a way that makes it easy to find the most similar vectors.
Example: Imagine you have a GRU processing text-based product descriptions, producing embeddings that capture each product’s characteristics. With Milvus, you can instantly retrieve related items for a new query—great for e-commerce platforms looking to offer fast and accurate product recommendations.
- Scalable and Efficient
Milvus can handle large-scale data (millions or billions of vectors) without sacrificing. performance.
Example: Suppose your GRU tracks user behavior in a subscription-based streaming service where each session generates a user preference embedding. As the platform grows, Milvus ensures these ever-expanding embeddings can be stored and retrieved quickly to keep pace with millions of daily active users.
- Real-Time Insights
Milvus is built to ingest data on the fly, so it can deliver insights the moment new vectors arrive.
Example: A GRU might embed network activity logs for a cybersecurity system to spot patterns linked to potential intrusions. As new logs stream in, those embeddings go straight into Milvus, allowing security teams to detect anomalies and address threats before they escalate.
Conclusion
GRUs capture patterns over long sequences without running into the severe gradient issues that affect basic RNNs. GRUs tackle the vanishing gradient problem in traditional RNNs by using gates that keep important information alive over time. They’re simpler and often faster to train than LSTMs, which makes them a popular choice for tasks like language modeling, time-series forecasting, and anomaly detection. Pairing GRUs with Milvus lets you store and query embeddings at a large scale for fast and accurate similarity searches, recommendations, and analytics. While newer architectures like Transformers are powerful, GRUs remain popular for many real-world applications.
FAQs on GRU
Do GRUs completely solve the vanishing gradient issue? They don’t eliminate it entirely, but their gating mechanism makes it much less severe than in basic RNNs.
Are GRUs always better than LSTMs? Not necessarily. GRUs have fewer parameters and can train faster, but LSTMs sometimes work better for very complex tasks. It depends on your data and goals.
Can GRUs handle very long sequences? They do better than simple RNNs, but extremely long sequences might still pose challenges. Transformers may be more suitable for tasks involving very long inputs.
How do GRUs work with Milvus? GRUs create vector embeddings of your sequence data. Milvus stores and indexes these embeddings, letting you do quick similarity searches and other vector-based queries on large datasets.
What are common GRU use cases? GRUs are used in text classification, speech recognition, recommendation systems, and sensor data analysis. Their efficiency and ease of use make them popular in real-time or resource-limited scenarios.
Related Resources
- Background: From RNNs to GRUs
- How Does GRU Work?
- GRU vs. LSTM: Key Differences
- Implementation in Python
- Use Cases & Applications
- Advantages of GRU
- Limitations of GRU
- Empowering GRUs with Milvus: The Perfect Match for Vector Search
- Conclusion
- FAQs on GRU
- Related Resources
Content
Start Free, Scale Easily
Try the fully-managed vector database built for your GenAI applications.
Try Zilliz Cloud for Free