Spring AI and Milvus: Using Milvus as a Spring AI Vector Store
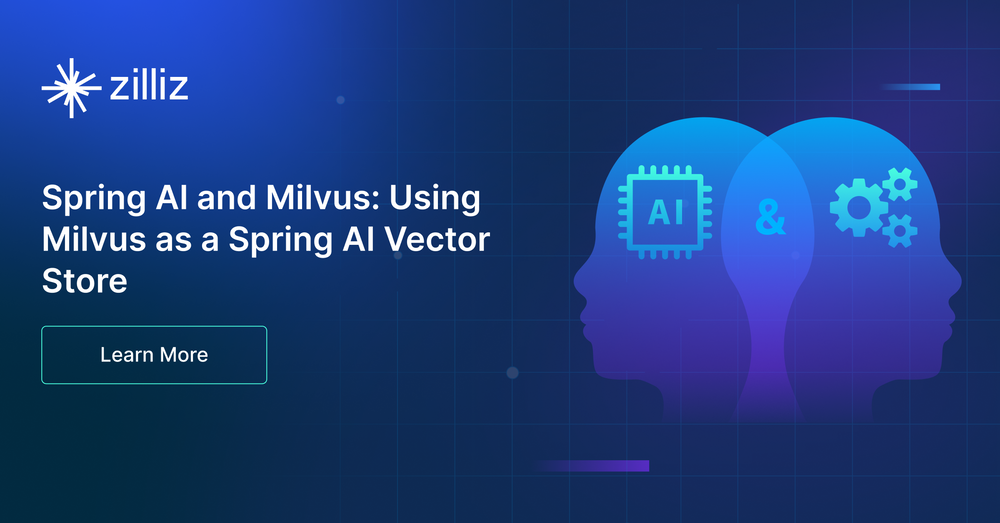
Introduction
In today's world, the ability to quickly process and analyze large volumes of data can significantly enhance the functionality of applications. This is particularly true in fields like artificial intelligence and machine learning, where data often exists in high-dimensional vector form. Integrating vector databases like Milvus into Spring AI applications addresses this need by providing efficient data handling and retrieval capabilities. This integration allows developers to perform complex queries and similarity searches quickly and accurately, facilitating better user experiences and more intelligent application behavior.
Milvus stands out in the vector database landscape due to its robust features tailored for scalability and performance in managing vector data. As an open-source platform, it offers a flexible and cost-effective solution for businesses and developers looking to leverage advanced application search capabilities. Milvus supports a variety of indexing strategies, which are crucial for optimizing search performance across large, unstructured documents. This makes it an excellent choice for applications involving image and video recognition, text analysis, and any other domain where similarity search is crucial.
Setup and Configuration for Integrating Milvus with Spring Boot Applications
Setting Up Milvus Using Docker Compose
To integrate Milvus with Spring AI in a Spring Boot application, start by setting up a Milvus instance using Docker Compose. This method is straightforward and suitable for development environments.
Procedure:
Download the Docker Compose YAML file: Use the following command to download the specific version of the Milvus Docker Compose file:
wget https://github.com/milvus-io/milvus/releases/download/v2.4.0/milvus-standalone-docker-compose.yml -O docker-compose.yml
Start the Milvus instance: Navigate to the directory containing the docker-compose.yml file and start the Milvus instance:
docker-compose up -d
Ensure that Docker Compose V2 is installed as it is recommended over V1 for better performance and compatibility. You can check for VectorDB’s presence on Docker Desktop(Windows) once the command is finished running:
For linux you can run this command:
docker ps
2. Create a Spring Boot Project
Generate the project: Use Spring Initializr to create a new Spring Boot project. Select either Maven or Gradle(The one used here was Gradle) as the build tool and add dependencies such as 'Spring Web' and 'Spring Data JPA'.
Setup the project environment: After downloading, extract the zip file and open the project in your preferred IDE.
Spring Initializr
3. Add Dependencies for Milvus and OpenAI Integration
Depending on whether you are using Maven or Gradle, add the necessary dependencies to integrate Milvus and OpenAI with Spring AI.
For Gradle (build.gradle):
repositories {
mavenCentral()
maven { url 'https://repo.spring.io/milestone' }
maven { url 'https://repo.spring.io/snapshot' }
}
dependencies {
implementation 'org.springframework.ai:spring-ai-milvus-store-spring-boot-starter:0.8.1-SNAPSHOT'
implementation 'org.springframework.ai:spring-ai-openai-spring-boot-starter'
implementation 'org.springframework.boot:spring-boot-starter-data-jpa'
implementation 'org.springframework.boot:spring-boot-starter-web'
// Other dependencies
}
Connecting Spring AI with Milvus: Detailed Configuration and Usage
In the context of Spring AI, Milvus serves as the backend for storing and managing vector data, allowing for rapid and scalable retrieval of similar items based on vector similarity. Here is how you can connect them together.
1. Configure Milvus and OpenAI in Spring Boot
Add the following properties to your application.properties or application.yml to configure the connection to Milvus and set up OpenAI embeddings:
For application.properties:
spring.application.name=demo
spring.autoconfigure.exclude=org.springframework.boot.autoconfigure.jdbc.DataSourceAutoConfiguration
spring.ai.vectorstore.milvus.client.host=localhost
spring.ai.vectorstore.milvus.client.port=19530
spring.ai.vectorstore.milvus.client.username=root
spring.ai.vectorstore.milvus.client.password=milvus
spring.ai.vectorstore.milvus.databaseName=default
spring.ai.vectorstore.milvus.collectionName=vector_store_1
spring.ai.vectorstore.milvus.embeddingDimension=1536
spring.ai.vectorstore.milvus.indexType=IVF_FLAT
spring.ai.vectorstore.milvus.metricType=COSINE
spring.ai.openai.api-key=YOUR_API_KEY
spring.ai.openai.embedding.options.model=text-embedding-ada-002
Replace YOUR_API_KEY with the actual API key obtained from OpenAI. This setup provides a basic configuration for integrating Milvus with a Spring Boot application using Spring AI and OpenAI for embeddings. Adjust the configurations and dependencies as necessary based on your specific requirements and environment.
2. Autowire the VectorStore in Your Service
In your Spring service, autowire the VectorStore to interact with the Milvus database. This is how it can be done:
package com.example.demo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.ai.vectorstore.VectorStore;
import org.springframework.ai.document.Document;
import org.springframework.ai.vectorstore.SearchRequest;
import java.util.List;
import java.util.Map;
@Service
public class VectorStoreService {
@Autowired
private VectorStore vectorStore;
}
3. Using the EmbeddingClient for Generating Embeddings
To generate embeddings that can be stored in Milvus, use the EmbeddingClient provided by Spring AI. This is how it can be done:
package com.example.demo.controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.ai.embedding.EmbeddingClient;
import org.springframework.ai.embedding.EmbeddingResponse;
import java.util.List;
import java.util.Map;
@RestController
public class EmbeddingController {
private final EmbeddingClient embeddingClient;
@Autowired
public EmbeddingController(EmbeddingClient embeddingClient) {
this.embeddingClient = embeddingClient;
}
}
Java Code Examples: Implementing Vector Storage
Storing and Retrieving Vector Data
To effectively utilize Milvus for vector storage within Spring AI applications, developers need to understand how to store, retrieve, and manage vector data. Below are Java code examples that demonstrate these functionalities.
Adding Documents to Milvus:
@Service
public class VectorStoreService {
@Autowired
private VectorStore vectorStore;
public void addDocuments() {
List<Document> documents = List.of(
new Document("Exploring the depths of AI with Spring", Map.of("category", "AI")),
new Document("Navigating through large datasets efficiently"),
new Document("Harnessing the power of vector search", Map.of("importance", "high"))
);
vectorStore.add(documents);
}
}
Searching for Similar Documents:
public List<Document> searchSimilarDocuments() {
return vectorStore.similaritySearch(
SearchRequest.query("Efficient data processing and retrieval")
.withTopK(3)
.withSimilarityThreshold(0.1)
);
}
Generating Embeddings with OpenAI:
Embeddings are crucial for vector-based searches. Here's how you can generate embeddings using OpenAI's service within a Spring AI application:
@RestController
public class EmbeddingController {
private final EmbeddingClient embeddingClient;
@Autowired
public EmbeddingController(EmbeddingClient embeddingClient) {
this.embeddingClient = embeddingClient;
}
@GetMapping("/ai/embedding")
public Map<String, Object> embed(@RequestParam(value = "message", defaultValue = "What's the latest in AI?") String message) {
EmbeddingResponse embeddingResponse = this.embeddingClient.embedForResponse(List.of(message));
return Map.of("embedding", embeddingResponse);
}
}
Advanced Configuration and Customization
Milvus offers several advanced settings for optimizing vector storage and retrieval. These include custom indexing strategies and performance tuning options.
Example of Advanced Configuration in Milvus:
spring.ai.vectorstore.milvus.indexType=HNSW
spring.ai.vectorstore.milvus.metricType=EUCLIDEAN
These properties can be set in the application.properties file to use different indexing strategies like Hierarchical Navigable Small World (HNSW) and different metric types such as EUCLIDEAN for distance calculation.
Use Cases and Practical Applications of Milvus in Spring AI
Recommendation Systems:
Milvus powers recommendation systems across various industries by storing user and item embeddings and performing similarity searches. This approach is particularly effective in personalized recommendations for movies, music, products, and content feeds. For instance, a movie recommendation system can use Milvus to match user preferences semantically with movie vectors, suggesting films that align with individual tastes without requiring explicit user queries. More on that here.
Content Search Engines: For applications involving large datasets of unstructured textual content, Milvus can transform text into vector form and perform efficient similarity searches with various methodologies. This capability is crucial for developing sophisticated search engines that go beyond keyword matching to understand the semantic context of user queries, thereby improving the relevance and accuracy of search results.
Challenges and Solutions
- Data Scalability
Handling vast amounts of vector data can be challenging. Milvus addresses this through features like partitioning and sharding, which help manage large datasets by distributing data across different nodes, thus enhancing performance and scalability.
- Query Latency
Optimizing index parameters and scaling the Milvus cluster effectively reduce query latency. Milvus supports various index types like HNSW, IVF, and Product Quantization, which can be tailored to specific use cases to balance speed and accuracy.
Advanced Use Cases
Image and Video Recognition
Milvus is used in high-throughput image and video analysis applications, such as video deduplication systems and image similarity searches. For example, platforms like Shopee utilize Milvus to enhance real-time video recall and copyright-matching search capabilities.
AI-Driven Chatbots and Customer Support
Integrating Milvus with AI models enables the development of RAG-based chatbots to understand and respond to user queries with high relevance and accuracy. Companies like PayPal and Farfetch use Milvus to power conversational AI for customer support and personalized shopping experiences.
Conclusion
Milvus significantly enhances the capabilities of Spring AI applications by providing efficient management and retrieval of vector data. Its versatility across various domains—e-commerce and entertainment to healthcare and financial services—demonstrates its potential to transform industries by enabling more intelligent, responsive, and personalized application functionalities. Developers are encouraged to leverage Milvus's advanced features and flexible configuration options to optimize their applications and explore new possibilities in AI-driven solutions.
- Introduction
- Setup and Configuration for Integrating Milvus with Spring Boot Applications
- Connecting Spring AI with Milvus: Detailed Configuration and Usage
- Java Code Examples: Implementing Vector Storage
- Use Cases and Practical Applications of Milvus in Spring AI
- Challenges and Solutions
- Advanced Use Cases
- Conclusion
Content
Start Free, Scale Easily
Try the fully-managed vector database built for your GenAI applications.
Try Zilliz Cloud for Free